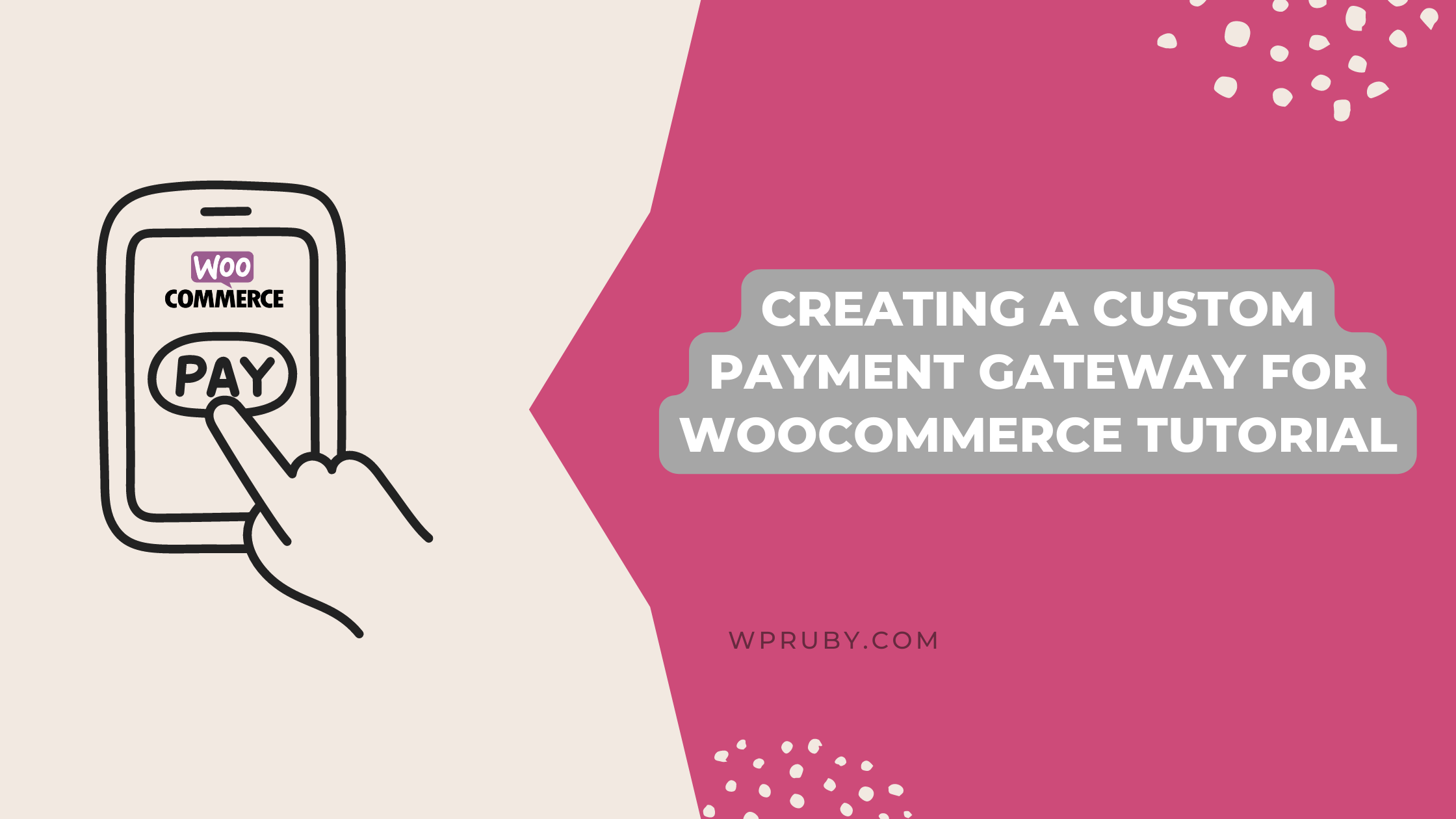
WooCommerce, the popular WordPress plugin, offers a robust platform for creating online stores. By integrating custom payment gateways, you can provide your customers with diverse payment options. In this guide, we’ll walk you through the process of creating a payment gateway extension for WooCommerce.
Prerequisites
- A WordPress installation with WooCommerce is already set up.
- Basic knowledge of PHP, WordPress development, and WooCommerce hooks.
- A local development environment or web hosting with WordPress installed.
Step 1: Create a New Plugin
- Start by creating a new folder within the
wp-content/plugins
directory of your WordPress installation. Name it something likecustom-payment-gateway
. - Inside the plugin folder, create a main PHP file (e.g.,
custom-payment-gateway.php
).
Step 2: Define the Plugin Basics
In custom-payment-gateway.php
, define the plugin details and initiate the plugin.
/*
Plugin Name: Custom Payment Gateway
Description: A custom payment gateway for WooCommerce.
Version: 1.0
Author: Your Name
*/
// Prevent direct access to this file
if (!defined(‘ABSPATH’)) {
exit;
}
// Initialize the plugin
function custom_payment_gateway_init() {
if (class_exists(‘WC_Payment_Gateway’)) {
include ‘class-custom-payment-gateway.php’; // Include your gateway class file
add_filter(‘woocommerce_payment_gateways’, ‘add_custom_payment_gateway’);
}
}
add_action(‘plugins_loaded’, ‘custom_payment_gateway_init’);
Step 3: Create the Payment Gateway Class
Create a new PHP file named class-custom-payment-gateway.php
in the same plugin directory. This class will extend the WC_Payment_Gateway
class provided by WooCommerce.
if (!defined(‘ABSPATH’)) {
exit;
}
class Custom_Payment_Gateway extends WC_Payment_Gateway {
public function __construct() {
// Define your payment gateway settings
$this->id = ‘custom_payment_gateway’;
$this->method_title = ‘Custom Payment Gateway’;
$this->method_description = ‘Pay using Custom Payment Gateway’;
$this->has_fields = true; // Set to true if you need custom payment fields
// Load settings
$this->init_form_fields();
$this->init_settings();
// Define gateway settings
$this->title = $this->get_option(‘title’);
$this->description = $this->get_option(‘description’);
// Add hooks
add_action(‘woocommerce_update_options_payment_gateways_’ . $this->id, array($this, ‘process_admin_options’));
}
// Define payment fields (if needed)
public function payment_fields() {
// Display custom payment fields here
}
// Process payment
public function process_payment($order_id) {
// Handle payment processing and return success or failure
}
}
Step 4: Display the Gateway Settings in WooCommerce Admin
In the class-custom-payment-gateway.php
file, add the following function to display the gateway settings in WooCommerce admin.
// Add gateway settings
public function init_form_fields() {
$this->form_fields = array(
‘enabled’ => array(
‘title’ => ‘Enable/Disable’,
‘type’ => ‘checkbox’,
‘label’ => ‘Enable Custom Payment Gateway’,
‘default’ => ‘no’,
),
‘title’ => array(
‘title’ => ‘Title’,
‘type’ => ‘text’,
‘description’ => ‘Title displayed during checkout.’,
‘default’ => ‘Custom Payment’,
‘desc_tip’ => true,
),
‘description’ => array(
‘title’ => ‘Description’,
‘type’ => ‘textarea’,
‘description’ => ‘Description displayed during checkout.’,
‘default’ => ‘Pay using our custom payment gateway.’,
),
);
}
Step 5: Test Your Payment Gateway
- Activate the plugin from the WordPress admin.
- Go to WooCommerce > Settings > Payments and enable your custom payment gateway.
- Configure the settings, including the title and description.
- Test the payment gateway in your store’s checkout process.
Conclusion
Remember, this is a simplified guide, and creating a fully functional payment gateway extension involves more details, such as handling payment responses, validating transactions, and ensuring security. Additionally, ensure your code adheres to WooCommerce and WordPress best practices.
When developing payment gateways, it’s crucial to follow security guidelines and thoroughly test your extension in a secure environment before deploying it to a live website. Always refer to the official WooCommerce and WordPress developer documentation for the latest information and coding practices.